Get QR code data from a png scalar
This example program demonstrates extracting the QR code data from a QR code generated with Image::PNG::QRCode using Image::PNG::Libpng and then writing it out again using Cairo. See also LeMoDa.net's Perl Cairo tutorial.
#!/home/ben/software/install/bin/perl use warnings; use strict; use utf8; use FindBin '$Bin'; use Image::PNG::QRCode 'qrpng'; use Image::PNG::Libpng ':all'; use Cairo; # Test getting the data from a QR code using Image::PNG::QRCode and # Image::PNG::Libpng. my $text = 'qrpng'; my $qrpng = qrpng (text => $text, scale => 1); my $png = read_from_scalar ($qrpng); my $rows = $png->get_rows (); my $header = $png->get_IHDR (); my $width = $header->{width}; my $height = $header->{height}; my @image; for my $row (@$rows) { my $bits = unpack ("B$width", $row); push @image, $bits; } my $scale = 5; my $surface = Cairo::ImageSurface->create ('argb32', $width * $scale, $height * $scale); my $cr = Cairo::Context->create ($surface); my $y = 0; my $x = 0; for my $row (@image) { for my $column (split //, $row) { if ($column) { $cr->set_source_rgb (1, 1, 1); } else { $cr->set_source_rgb (0, 0, 0); } $cr->rectangle ($x * $scale, $y * $scale, ($x + 1) * $scale, ($y + 1) * $scale); $cr->fill (); $x++; } $x = 0; $y++; } $surface->write_to_png ('qr.png');
The output looks like this:
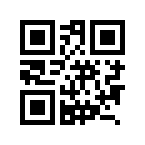
Copyright © Ben Bullock 2009-2023. All
rights reserved.
For comments, questions, and corrections, please email
Ben Bullock
(benkasminbullock@gmail.com) or use the discussion group at Google Groups.
/
Privacy /
Disclaimer