Compare strings ignoring case in C
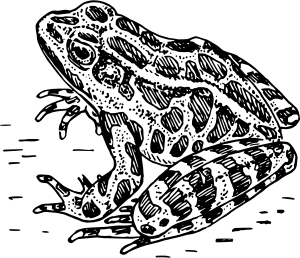
This is an example C program which illustrates comparing strings
with strcmp
and strcasecmp
. The latter
routine compares strings case-insensitively.
#include <stdio.h> #include <string.h> int main () { char * a = "BINGO"; char * b = "bingo"; /* Compare using strcmp. */ printf ("%s and %s are ", a, b); if (strcmp (a, b) == 0) { printf ("the same"); } else { printf ("different"); } printf (" according to 'strcmp';\nthey are "); if (strcasecmp (a, b) == 0) { printf ("the same"); } else { printf ("different"); } printf (" according to 'strcasecmp'.\n"); return 0; }
The output of the example looks like this:
BINGO and bingo are different according to 'strcmp'; they are the same according to 'strcasecmp'.
Copyright © Ben Bullock 2009-2024. All
rights reserved.
For comments, questions, and corrections, please email
Ben Bullock
(benkasminbullock@gmail.com).
/
Privacy /
Disclaimer