C macros __LINE__, __FILE__ and __func__
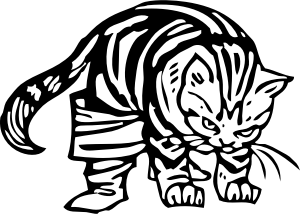
It is possible for a C program to print the currently executing line of source code, the file of the source code, and the name of the current function. The currently executing line is available in a preprocessor variable called __LINE__
:
#include <stdio.h> int main () { printf ("This is line %d.\n", __LINE__); return 0; }This prints out
This is line 5.
To get the name of the file, use the preprocessor variable __FILE__
:
#include <stdio.h> int main () { printf ("This is line %d of file \"%s\".\n", __LINE__, __FILE__); return 0; }This prints out
This is line 6 of file "/usr/home/ben/lemoda/c/line-file-func/file.c".
C99 (the most recent version of the C language, at the time of writing) introduced another macro called __func__
which also names the function in use:
#include <stdio.h> #define BLURT printf ("This is line %d of file %s (function %s)\n",\ __LINE__, __FILE__, __func__) void silly_function () { BLURT; } int main () { BLURT; silly_function (); return 0; }This prints out
This is line 13 of file /usr/home/ben/lemoda/c/line-file-func/func.c (function main) This is line 8 of file /usr/home/ben/lemoda/c/line-file-func/func.c (function silly_function)
You can change the values used for __FILE__
and __LINE__
using a line directive, which is a preprocessor command of the form
#line 20 "some-file"
which tells the C compiler that the next line after the line directive is line 20 of some-file
.
#include <stdio.h> #define BLURT printf ("This is line %d of file \"%s\" (function <%s>)\n",\ __LINE__, __FILE__, __func__) #line 99 "grody-to-the-max" void silly_function () { BLURT; } #line 999 "bassetts-liquorice-allsorts" int main () { BLURT; silly_function (); return 0; }This prints out
This is line 1001 of file "bassetts-liquorice-allsorts" (function <main>) This is line 101 of file "grody-to-the-max" (function <silly_function>)
This line directive is used by facilities like "lex" and "yacc" which output C code so that error messages are related to the input file rather than the output C file.
GCC (the "Gnu Compiler Collection") also
defines __FUNCTION__
and __PRETTY_FUNCTION__
. These are both the same
as __func__
for C. For
C++, __PRETTY_FUNCTION__
is something different.