An example of array initialization in C
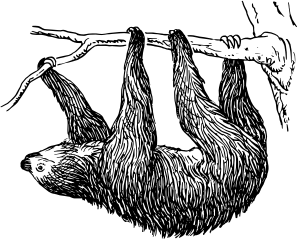
This example program illustrates array initialization in C.
#include <stdio.h> #define ARRAY_SIZE 10 int main () { int i; /* Initialize all the elements. */ int array_all[ARRAY_SIZE] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; /* If only the first elements are initialized, the remaining elements are all set to zero. */ int array_first_two[ARRAY_SIZE] = { 1, 2 }; /* So, if the first element is set to zero, then everything else is, too. */ int array_zero[ARRAY_SIZE] = { 0 }; /* But if nothing is initialized, the whole array contains random junk. */ int array_not_static[ARRAY_SIZE]; /* Unless the array is declared using "static", in which case it is zeroed. */ static int array_static[ARRAY_SIZE]; /* This prints out the contents of the arrays. */ for (i = 0; i < ARRAY_SIZE; i++) { printf ("%2d: %2d %d %d %12d %d\n", i, array_all[i], array_first_two[i], array_zero[i], array_not_static[i], array_static[i] ); } return 0; }
It outputs something like
0: 1 1 0 671490048 0 1: 2 2 0 134519028 0 2: 3 0 0 672654592 0 3: 4 0 0 671478452 0 4: 5 0 0 -1077942112 0 5: 6 0 0 671401671 0 6: 7 0 0 671481716 0 7: 8 0 0 -1077942192 0 8: 9 0 0 -1077942136 0 9: 10 0 0 1 0
The numbers in the second-to-last column will vary each time it's run.
Copyright © Ben Bullock 2009-2025. All
rights reserved.
For comments, questions, and corrections, please email
Ben Bullock
(benkasminbullock@gmail.com).
/
Privacy /
Disclaimer