Length of array in C
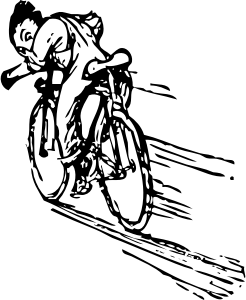
This example program demonstrates how to get the length of a C array
using the sizeof
operator.
#include <stdio.h> int int_array[5]; double double_array[10]; char char_array[] = {'a', 'b', 'c', 'd'}; int main () { const char * format = "The %s array has %d bytes and %d elements.\n"; printf (format, "int", sizeof (int_array), sizeof (int_array) / sizeof (int)); printf (format, "double", sizeof (double_array), sizeof (double_array) / sizeof (double)); /* This method works even when the size of the array is not specified in the initializer. */ printf (format, "char", sizeof (char_array), sizeof (char_array) / sizeof (char)); return 0; }
It outputs the following:
The int array has 20 bytes and 5 elements. The double array has 80 bytes and 10 elements. The char array has 4 bytes and 4 elements.
Copyright © Ben Bullock 2009-2024. All
rights reserved.
For comments, questions, and corrections, please email
Ben Bullock
(benkasminbullock@gmail.com).
/
Privacy /
Disclaimer