An example of Cairo and FreeType in C
This is an example C program showing the use of the Cairo graphics library and the FreeType library together to load an arbitrary type and display some text.
#include <stdio.h> #include <stdlib.h> #include <cairo.h> #include <cairo-ft.h> #include <ft2build.h> #include FT_SFNT_NAMES_H #include FT_FREETYPE_H #include FT_GLYPH_H #include FT_OUTLINE_H #include FT_BBOX_H #include FT_TYPE1_TABLES_H int main () { FT_Library value; FT_Error status; FT_Face face; cairo_t *c; cairo_surface_t *cs; cairo_font_face_t * ct; int size = 200; cs = cairo_image_surface_create (CAIRO_FORMAT_ARGB32, size, size); c = cairo_create (cs); const char * filename = "/usr/local/share/fonts/Droid/DroidSansFallbackFull.ttf"; status = FT_Init_FreeType (& value); if (status != 0) { fprintf (stderr, "Error %d opening library.\n", status); exit (EXIT_FAILURE); } status = FT_New_Face (value, filename, 0, & face); if (status != 0) { fprintf (stderr, "Error %d opening %s.\n", status, filename); exit (EXIT_FAILURE); } ct = cairo_ft_font_face_create_for_ft_face (face, 0); cairo_set_font_face (c, ct); cairo_set_font_size (c, size/3); cairo_set_source_rgb (c, 0, 0, 0); cairo_move_to (c, size/6, size/3); cairo_show_text (c, "あい"); cairo_set_source_rgb (c, 0.5, 0.5, 0); cairo_move_to (c, size/6, 5*size/6); cairo_show_text (c, "金柑"); cairo_surface_write_to_png (cs, "free-type.png"); return 0; }
The output of the example looks like this:
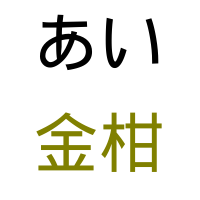
Here is a Makefile, which relies on the use of pkg-config
to
access the Cairo and FreeType libraries:
C=free-type.c CFLAGS = -Wall -g `pkg-config --cflags freetype2 cairo` LDFLAGS = `pkg-config --libs freetype2 cairo` all: free-type.png free-type.png: free-type ./free-type free-type: $(C) $(CC) $(CFLAGS) $(LDFLAGS) -o $@ $(C) clean: -rm -f free-type free-type.png
Copyright © Ben Bullock 2009-2025. All
rights reserved.
For comments, questions, and corrections, please email
Ben Bullock
(benkasminbullock@gmail.com).
/
Privacy /
Disclaimer