Draw a coloured graph of three phases
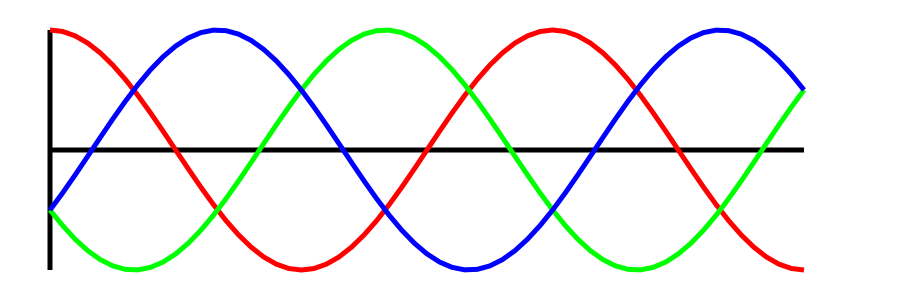
#!/home/ben/software/install/bin/perl # This program draws a graph of three phase electrical voltages. use warnings; use strict; use utf8; use FindBin '$Bin'; use Cairo; use constant PI => 3.14159; my $xscale = 80; my $yscale = 120; my $xoffset = 50; my $yoffset = 150; my $gap = 20; my $xmax = 3*PI; main (); sub main { my $ysize = 300; my $xsize = $ysize * 3; my $surface = Cairo::ImageSurface->create ('argb32', $xsize, $ysize); my $cr = Cairo::Context->create ($surface); $cr->set_line_width (5.); draw_axes ($cr); draw_cos ($cr, [1,0,0],0); draw_cos ($cr, [0,1,0],2*PI/3); draw_cos ($cr, [0,0,1],4*PI/3); $surface->write_to_png ("$Bin/three-phase.png"); } sub draw_axes { my ($cr) = @_; $cr->set_source_rgb (0, 0, 0); $cr->move_to ($xoffset, $yoffset); $cr->line_to ($xoffset + $xscale * $xmax, $yoffset); $cr->stroke (); $cr->move_to ($xoffset, $yoffset - $yscale); $cr->line_to ($xoffset, $yoffset + $yscale); $cr->stroke (); } sub draw_cos { my ($cr, $colour, $offset) = @_; $cr->set_source_rgb (@$colour); my $x = 0; $cr->move_to (point ($x, $offset)); while ($x < $xmax) { $x += PI/$gap; $cr->line_to (point ($x, $offset)); } $cr->stroke (); } sub point { my ($x, $offset) = @_; my $y = wye ($x, $offset); return ($x * $xscale + $xoffset, -$y * $yscale + $yoffset); } sub wye { my ($x, $offset) = @_; return cos ($x + $offset); }
Copyright © Ben Bullock 2009-2024. All
rights reserved.
For comments, questions, and corrections, please email
Ben Bullock
(benkasminbullock@gmail.com).
/
Privacy /
Disclaimer